JavaFx Sqlite Database Tutorial 5 - How to show and hide a window in Jav...
package application;
import java.io.IOException;
import java.net.URL;
import java.sql.SQLException;
import java.util.ResourceBundle;
import javafx.event.ActionEvent;
import javafx.fxml.FXML;
import javafx.fxml.FXMLLoader;
import javafx.fxml.Initializable;
import javafx.scene.Node;
import javafx.scene.Parent;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.control.TextField;
import javafx.scene.layout.Pane;
import javafx.stage.Stage;
public class LoginController implements Initializable {
public LoginModel loginModel = new LoginModel();
@FXML
private Label isConnected;
@FXML
private TextField txtUsername;
@FXML
private TextField txtPassword;
@Override
public void initialize(URL location, ResourceBundle resources) {
// TODO Auto-generated method stub
if (loginModel.isDbConnected()) {
isConnected.setText("Connected");
} else {
isConnected.setText("Not Connected");
}
}
public void Login (ActionEvent event) {
try {
if (loginModel.isLogin(txtUsername.getText(), txtPassword.getText())) {
isConnected.setText("username and password is correct");
((Node)event.getSource()).getScene().getWindow().hide();
Stage primaryStage = new Stage();
FXMLLoader loader = new FXMLLoader();
Pane root = loader.load(getClass().getResource("/application/User.fxml").openStream());
UserController userController = (UserController)loader.getController();
userController.GetUser(txtUsername.getText());
Scene scene = new Scene(root);
scene.getStylesheets().add(getClass().getResource("application.css").toExternalForm());
primaryStage.setScene(scene);
primaryStage.show();
} else {
isConnected.setText("username and password is not correct");
}
} catch (SQLException e) {
isConnected.setText("username and password is not correct");
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
package application;
import java.sql.*;
public class LoginModel {
Connection conection;
public LoginModel () {
conection = SqliteConnection.Connector();
if (conection == null) {
System.out.println("connection not successful");
System.exit(1);}
}
public boolean isDbConnected() {
try {
return !conection.isClosed();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
return false;
}
}
public boolean isLogin(String user, String pass) throws SQLException {
PreparedStatement preparedStatement = null;
ResultSet resultSet = null;
String query = "select * from employee where username = ? and password = ?";
try {
preparedStatement = conection.prepareStatement(query);
preparedStatement.setString(1, user);
preparedStatement.setString(2, pass);
resultSet = preparedStatement.executeQuery();
if (resultSet.next()) {
return true;
}
else {
return false;
}
} catch (Exception e) {
return false;
// TODO: handle exception
} finally {
preparedStatement.close();
resultSet.close();
}
}
}
package application;
import javafx.application.Application;
import javafx.fxml.FXMLLoader;
import javafx.stage.Stage;
import javafx.scene.Parent;
import javafx.scene.Scene;
import javafx.scene.layout.BorderPane;
public class Main extends Application {
@Override
public void start(Stage primaryStage) {
try {
Parent root = FXMLLoader.load(getClass().getResource("/application/Login.fxml"));
Scene scene = new Scene(root);
scene.getStylesheets().add(getClass().getResource("application.css").toExternalForm());
primaryStage.setScene(scene);
primaryStage.show();
} catch(Exception e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
launch(args);
}
}
package application;
import java.sql.*;
public class SqliteConnection {
public static Connection Connector() {
try {
Class.forName("org.sqlite.JDBC");
Connection conn =DriverManager.getConnection("jdbc:sqlite:EmployeeDb.sqlite");
return conn;
} catch (Exception e) {
System.out.println(e);
return null;
// TODO: handle exception
}
}
}
package application;
import java.net.URL;
import java.util.ResourceBundle;
import javafx.event.ActionEvent;
import javafx.fxml.FXML;
import javafx.fxml.FXMLLoader;
import javafx.fxml.Initializable;
import javafx.scene.Node;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.layout.Pane;
import javafx.stage.Stage;
public class UserController implements Initializable {
@FXML
private Label userLbl;
@Override
public void initialize(URL location, ResourceBundle resources) {
// TODO Auto-generated method stub
}
public void GetUser(String user) {
// TODO Auto-generated method stub
userLbl.setText(user);
}
public void SignOut(ActionEvent event) {
try {
((Node)event.getSource()).getScene().getWindow().hide();
Stage primaryStage = new Stage();
FXMLLoader loader = new FXMLLoader();
Pane root = loader.load(getClass().getResource("/application/Login.fxml").openStream());
Scene scene = new Scene(root);
scene.getStylesheets().add(getClass().getResource("application.css").toExternalForm());
primaryStage.setScene(scene);
primaryStage.show();
} catch (Exception e) {
// TODO: handle exception
}
}
}
<?xml version="1.0" encoding="UTF-8"?>
<?import javafx.scene.text.*?>
<?import javafx.scene.control.*?>
<?import java.lang.*?>
<?import javafx.scene.layout.*?>
<?import javafx.scene.layout.AnchorPane?>
<AnchorPane prefHeight="302.0" prefWidth="367.0" xmlns="http://javafx.com/javafx/8.0.40" xmlns:fx="http://javafx.com/fxml/1" fx:controller="application.LoginController">
<children>
<Label fx:id="isConnected" layoutY="35.0" prefHeight="46.0" prefWidth="367.0" text="Status" textFill="#cd1313">
<font>
<Font size="18.0" />
</font>
</Label>
<TextField fx:id="txtUsername" layoutX="54.0" layoutY="108.0" promptText="User Name">
<font>
<Font size="18.0" />
</font>
</TextField>
<PasswordField fx:id="txtPassword" layoutX="54.0" layoutY="157.0" promptText="Password">
<font>
<Font size="18.0" />
</font>
</PasswordField>
<Button layoutX="103.0" layoutY="216.0" mnemonicParsing="false" onAction="#Login" text="Login">
<font>
<Font size="18.0" />
</font>
</Button>
</children>
</AnchorPane>
<?xml version="1.0" encoding="UTF-8"?>
<?import javafx.scene.control.*?>
<?import java.lang.*?>
<?import javafx.scene.layout.*?>
<?import javafx.scene.layout.AnchorPane?>
<BorderPane maxHeight="-Infinity" maxWidth="-Infinity" minHeight="-Infinity" minWidth="-Infinity" prefHeight="400.0" prefWidth="600.0" xmlns="http://javafx.com/javafx/8.0.40" xmlns:fx="http://javafx.com/fxml/1" fx:controller="application.UserController">
<top>
<HBox BorderPane.alignment="CENTER">
<children>
<Label fx:id="userLbl" prefHeight="34.0" prefWidth="106.0" text="User Welcome" />
<Button mnemonicParsing="false" onAction="#SignOut" prefHeight="28.0" prefWidth="103.0" text="Sign Out" />
</children>
</HBox>
</top>
</BorderPane>
JavaFx Sqlite Database Tutorial 5 - How to show and hide a window in Jav...
Reviewed by Mursal Zheker
on
Selasa, November 24, 2015
Rating:
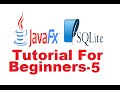
Tidak ada komentar: